Using Stylify CSS in Svelte
Svelte is a radical new approach to building user interfaces. Whereas traditional frameworks like React and Vue do the bulk of their work in the browser, Svelte shifts that work into a compile step that happens when you build your app.
You can use Stylify CSS with SvelteKit or with Vite + Svelte App. Below are guides for both scenarios.
How to integrate the Stylify CSS with SvelteKit
Try it on StackBlitz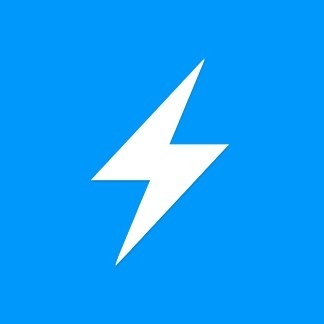
First, install the @stylify/unplugin package using NPM or Yarn:
npm i -D @stylify/unplugin
yarn add -D @stylify/unplugin
Next add the following configuration into the vite.config.js
:
import { sveltekit } from '@sveltejs/kit/vite';
import { stylifyVite } from '@stylify/unplugin';
const stylifyPlugin = stylifyVite({
bundles: [{
outputFile: './src/stylify.css',
files: ['./src/**/*.{svelte,html}'],
}]
});
const config = {
plugins: [
stylifyPlugin,
sveltekit(),
]
};
export default config;
The last step, create src/routes/+layout.svelte
with the following content stylify.css
:
<script>
import '../stylify.css';
</script>
<slot />
In case, you have created more bundles, for example for pages, you have to add paths to those generated CSS files into correct Svelte files.
Now run yarn dev
. The src/stylify.css
file will be generated.
How to integrate the Stylify CSS with Svelte and Vite.js
Try it on StackBlitz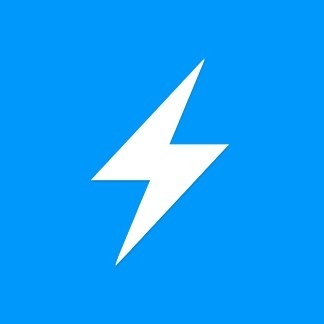
The example below works with the Vite - Svelte template. You can however use the example below and configure it for React.js, Vue and any other framework you use.
First, install the @stylify/unplugin package using NPM or Yarn:
npm i -D @stylify/unplugin
yarn add -D @stylify/unplugin
Next add the following configuration into the vite.config.js
:
import { defineConfig } from 'vite'
import { svelte } from '@sveltejs/vite-plugin-svelte'
import { stylifyVite } from '@stylify/unplugin';
const stylifyPlugin = stylifyVite({
bundles: [{ outputFile: './src/stylify.css', files: ['./src/**/*.svelte'] }],
// Optional
// Compiler config info https://stylifycss.com/en/docs/stylify/compiler#configuration
compiler: {
// https://stylifycss.com/en/docs/stylify/compiler#variables
variables: {},
// https://stylifycss.com/en/docs/stylify/compiler#macros
macros: {},
// https://stylifycss.com/en/docs/stylify/compiler#components
components: {},
// ...
}
});
export default defineConfig({
plugins: [stylifyPlugin, svelte()]
});
Now you can add the path of the generated src/stylify.css
into src/main.js
file:
import './stylify.css';
Now run yarn dev
. The src/stylify.css
file will be generated.
Configuration
There is a lot of options you can configure:
Other tips
In case you use the Stylify group syntax like [h1,h2]{color:blue}
, svelte will match the \{color
as variable. To avoid that, use the syntax with curly brackets and template literals for class attributes:
class={` [h1,h2]{color:blue} `}
Where to go next?
- 🚀 Learn how to get started
- 🔌 Check out @stylify/unplugin configuration
- ⚙️ Or configure Stylify right away: